§¹???:
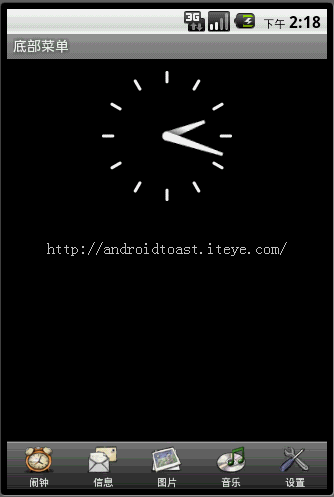
??????????????
Java????
-
<?xml version="1.0" encoding="UTF-8"?>
-
<TabHost android:id="@android:id/tabhost" android:layout_width="fill_parent" android:layout_height="fill_parent"
-
xmlns:android="http://schemas.android.com/apk/res/android">
-
<LinearLayout
-
android:orientation="vertical"
-
android:layout_width="fill_parent"
-
android:layout_height="fill_parent">
-
<FrameLayout
-
android:id="@android:id/tabcontent"
-
android:layout_width="fill_parent"
-
android:layout_height="0.0dip"
-
android:layout_weight="1.0" />
-
<TabWidget
-
android:id="@android:id/tabs"
-
android:visibility="gone"
-
android:layout_width="fill_parent"
-
android:layout_height="wrap_content"
-
android:layout_weight="0.0" />
-
<RadioGroup
-
android:gravity="center_vertical"
-
android:layout_gravity="bottom"
-
android:orientation="horizontal"
-
android:id="@+id/main_radio"
-
android:background="@drawable/maintab_toolbar_bg"
-
android:layout_width="fill_parent"
-
android:layout_height="wrap_content">
-
<RadioButton
-
android:id="@+id/radio_button0"
-
android:tag="radio_button0"
-
android:layout_marginTop="2.0dip"
-
android:text="@string/alarm"
-
android:drawableTop="@drawable/icon_1"
-
style="@style/main_tab_bottom" />
-
<RadioButton
-
android:id="@+id/radio_button1"
-
android:tag="radio_button1"
-
android:layout_marginTop="2.0dip"
-
android:text="@string/message"
-
android:drawableTop="@drawable/icon_2"
-
style="@style/main_tab_bottom" />
-
<RadioButton
-
android:id="@+id/radio_button2"
-
android:tag="radio_button2"
-
android:layout_marginTop="2.0dip"
-
android:text="@string/photo"
-
android:drawableTop="@drawable/icon_3"
-
style="@style/main_tab_bottom" />
-
<RadioButton
-
android:id="@+id/radio_button3"
-
android:tag="radio_button3"
-
android:layout_marginTop="2.0dip"
-
android:text="@string/music"
-
android:drawableTop="@drawable/icon_4"
-
style="@style/main_tab_bottom" />
-
<RadioButton
-
android:id="@+id/radio_button4"
-
android:tag="radio_button4"
-
android:layout_marginTop="2.0dip"
-
android:text="@string/setting"
-
android:drawableTop="@drawable/icon_5"
-
style="@style/main_tab_bottom" />
-
</RadioGroup>
-
</LinearLayout>
-
</TabHost>
???????????????TabHost?????????????§Þ???ID????????§Õ???android:id="@android:id/tabhost ??android:id="@android:id/tabcontent" ??android:id="@android:id/tabs" ???????????§Õ???????TabHost??????§³????????????????ID???????????
??TabActivity?????????????
Java??????????????????????????????????????§Ò????????????????????????????????TabHost????????????ID?tabhost???????
?????TabHost??????§µ?
Java????
-
public void setup() {
-
mTabWidget = (TabWidget) findViewById(com.android.internal.R.id.tabs);
-
if (mTabWidget == null) {
-
throw new RuntimeException(
-
"Your TabHost must have a TabWidget whose id attribute is 'android.R.id.tabs'");
-
}
-
-
// KeyListener to attach to all tabs. Detects non-navigation keys
-
// and relays them to the tab content.
-
mTabKeyListener = new OnKeyListener() {
-
public boolean onKey(View v, int keyCode, KeyEvent event) {
-
switch (keyCode) {
-
case KeyEvent.KEYCODE_DPAD_CENTER:
-
case KeyEvent.KEYCODE_DPAD_LEFT:
-
case KeyEvent.KEYCODE_DPAD_RIGHT:
-
case KeyEvent.KEYCODE_DPAD_UP:
-
case KeyEvent.KEYCODE_DPAD_DOWN:
-
case KeyEvent.KEYCODE_ENTER:
-
return false;
-
-
}
-
mTabContent.requestFocus(View.FOCUS_FORWARD);
-
return mTabContent.dispatchKeyEvent(event);
-
}
-
-
};
-
-
mTabWidget.setTabSelectionListener(new TabWidget.OnTabSelectionChanged() {
-
public void onTabSelectionChanged(int tabIndex, boolean clicked) {
-
setCurrentTab(tabIndex);
-
if (clicked) {
-
mTabContent.requestFocus(View.FOCUS_FORWARD);
-
}
-
}
-
});
-
-
mTabContent = (FrameLayout) findViewById(com.android.internal.R.id.tabcontent);
-
if (mTabContent == null) {
-
throw new RuntimeException(
-
"Your TabHost must have a FrameLayout whose id attribute is "
-
+ "'android.R.id.tabcontent'");
-
}
-
}
???????
?????????????????????????????¨¢??????????????????tabs ???ID????????TabWidget?????tabcontent???ID????????FrameLayout????????????????????????????ID????????
???????????????????????????Widget???????????????????Button??
????????????
Java????
-
package com.iteye.androidtoast;
-
-
import android.app.TabActivity;
-
import android.content.Intent;
-
import android.os.Bundle;
-
import android.widget.RadioGroup;
-
import android.widget.RadioGroup.OnCheckedChangeListener;
-
import android.widget.TabHost;
-
-
public class MainActivity extends TabActivity implements OnCheckedChangeListener{
-
/** Called when the activity is first created. */
-
private TabHost mHost;
-
private RadioGroup radioderGroup;
-
@Override
-
public void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
setContentView(R.layout.maintabs);
-
//?????TabHost
-
mHost=this.getTabHost();
-
-
//??????
-
mHost.addTab(mHost.newTabSpec("ONE").setIndicator("ONE")
-
.setContent(new Intent(this,OneActivity.class)));
-
mHost.addTab(mHost.newTabSpec("TWO").setIndicator("TWO")
-
.setContent(new Intent(this,TwoActivity.class)));
-
mHost.addTab(mHost.newTabSpec("THREE").setIndicator("THREE")
-
.setContent(new Intent(this,ThreeActivity.class)));
-
mHost.addTab(mHost.newTabSpec("FOUR").setIndicator("FOUR")
-
.setContent(new Intent(this,FourActivity.class)));
-
mHost.addTab(mHost.newTabSpec("FIVE").setIndicator("FIVE")
-
.setContent(new Intent(this,FiveActivity.class)));
-
-
radioderGroup = (RadioGroup) findViewById(R.id.main_radio);
-
radioderGroup.setOnCheckedChangeListener(this);
-
}
-
@Override
-
public void onCheckedChanged(RadioGroup group, int checkedId) {
-
switch(checkedId){
-
case R.id.radio_button0:
-
mHost.setCurrentTabByTag("ONE");
-
break;
-
case R.id.radio_button1:
-
mHost.setCurrentTabByTag("TWO");
-
break;
-
case R.id.radio_button2:
-
mHost.setCurrentTabByTag("THREE");
-
break;
-
case R.id.radio_button3:
-
mHost.setCurrentTabByTag("FOUR");
-
break;
-
case R.id.radio_button4:
-
mHost.setCurrentTabByTag("FIVE");
-
break;
-
}
-
}
-
}
|